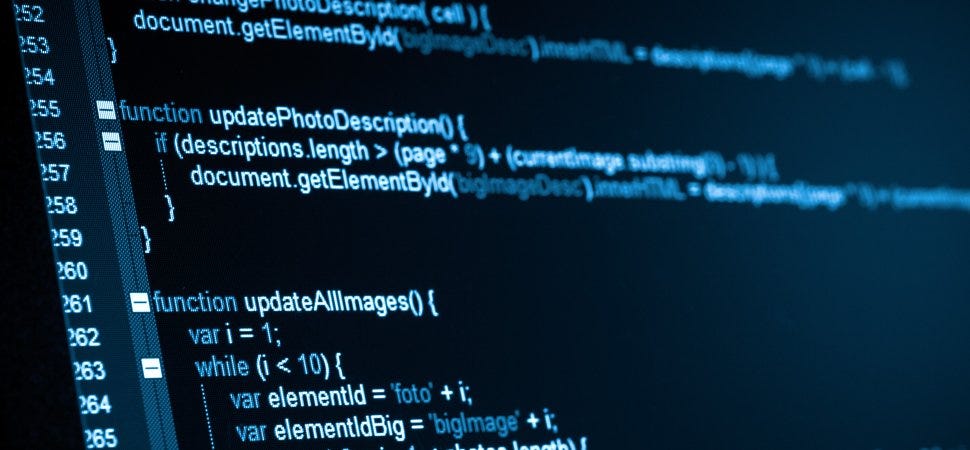
The 2019 edition of the ECMAScript specification has many new features. Among them, I will summarize the ones that seem most useful to me.
First, you can run these examples in node.js ≥12. To Install Node.js 12 on Ubuntu-Debian-Mint you can do the following:
$sudo apt update
$sudo apt -y upgrade
$sudo apt update
$sudo apt -y install curl dirmngr apt-transport-https lsb-release ca-certificates
$curl -sL https://deb.nodesource.com/setup_12.x | sudo -E bash -
$sudo apt -y install nodejs
Or, in Chrome Version ≥72, you can try those features in the developer console(Alt +j).
Array.prototype.flat && Array.prototype. flatMap
The flat() method creates a new array with all sub-array elements concatenated into it recursively up to the specified depth.
let array1 = ['a','b', [1, 2, 3]];
let array2 = array1.flat();
//['a', 'b', 1, 2, 3]
We should also note that the method excludes gaps or empty elements in the array:
let array1 = ['a', 'b', , , , 'c'];
let array2 = array1.flat();
// ['a','b','c']
The flatMap() method has the same effect as using the map() method followed by the flat() method with the default depth of 1
let array1 = [1, 2, 3, 4];array1.flatMap(x => [x + 1]); // [2, 3, 4, 5]
Object.fromEntries()
It creates an object or transforms key-value pairs into an object. It only accepts iterables.
const obj = { a: 1, b: 2, c: 3};const entries = Object.entries(obj); // entries (3) [Array(2), Array(2), Array(2)] 0: (2) ["a", 1] 1: (2) ["b", 2] 2: (2) ["c", 3]const obj2 = Object.fromEntries(entries) // {a: 1, b: 2, c: 3}
String.trimStart() & String.trimEnd()
The trimStart()/trimEnd() methods removes whitespace from the beginning/end of a string.
let result = ' hello!'.trimStart(); // "hello!"let cadena = 'hello '.trimEnd() // "hello!"
Optional Catch Binding
It allows us to use try /catch without having supplied the error parameter within the catch block.
Without optional catch binding:
try {
// do something
} catch (e) {
// we have error parameter to handle it
}
With optional catch binding:
try {
// do something
} catch {
// no binding or parameter to handle it
}
Although it is not a recommended practice in most cases, it makes sense when you know you’re not going to use the exception object.
Function “toString” Revision
Now the toString method must return source text slice from the beginning of the first token to end of the last token matched by the appropriate grammar.
function /* kk*/ foo() { /* cc */ }// before: foo.toString(); // "function foo() {}"// Now: foo.toString(); //"function /* kk*/ foo() { /* cc */ }"
Array.Sort Stability
Previously, V8 used an unstable QuickSort for arrays with more than 10 elements. V8(Chrome ≥70) uses the stable TimSort algorithm.
const array1 = [ { name: "a", age: 14 }, { name: "b", age: 14 }, { name: "c", age: 13 }, { name: "d", age: 13 }, { name: "e", age: 13 }, { name: "f", age: 13 }, { name: "g", age: 13 }, { name: "h", age: 13 }, { name: "i", age: 12 }, { name: "j", age: 12 }, { name: "k", age: 12 } ]const array2 = array1.sort( (a,b) => b.age - a.age)
The array is pre-sorted alphabetically by name. To sort by age, we pass a custom callback that compares the ages. This is the result that you’d expect:
0: {name: "a", age: 14}
1: {name: "b", age: 14}
2: {name: "c", age: 13}
3: {name: "d", age: 13}
4: {name: "e", age: 13}
5: {name: "f", age: 13}
6: {name: "g", age: 13}
7: {name: "h", age: 13}
8: {name: "i", age: 12}
9: {name: "j", age: 12}
10: {name: "k", age: 12}
The array is sorted by age, but within each age, they’re still sorted alphabetically by name.
Before, the JavaScript specification didn’t require sort stability for Array.sort, and instead left it up to the implementation. And because this you could also get this sort of result where “b” appears before “a”:
0: {name: "b", age: 14}
1: {name: "a", age: 14}
2: {name: "c", age: 13}
3: {name: "d", age: 13}
4: {name: "e", age: 13}
5: {name: "f", age: 13}
6: {name: "g", age: 13}
7: {name: "h", age: 13}
8: {name: "i", age: 12}
9: {name: "j", age: 12}
10: {name: "k", age: 12}
Symbol.description
Symbol is a built-in datatype for unique identifiers. Now a new property Symbol.prototype.description is added to get a description from symbol.
Before
const symbol1 = Symbol('my symbol');
console.log(symbol1.toString());//Symbol(my symbol)
Now:
const symbol1 = Symbol('my symbol'); console.log(symbol1) console.log(String(symbol1) === `Symbol(${'my symbol'})`); console.log(symbol1.description);//Symbol(my symbol) //true //my symbol
Comments
Post a Comment