What Is Auth0?
Auth0 is a third-party tool that provide services for authentication and authorization. It provides the building blocks for authentication and authorization, allowing you to secure your applications without having to become security experts. You can integrate Auth0 into any Node.js app.
To implement Auth0 with Node.js, first you need to create an account on Auth0. Then, log in.
Step 1: Get the Auth0 Application Keys
Now we need to do some configuration on Auth0, so let’s do it.
When you signed up for Auth0, a new application was created for you — or you could have created a new one. We need the following information:
- Domain key
- Client ID key
- Client-secret key
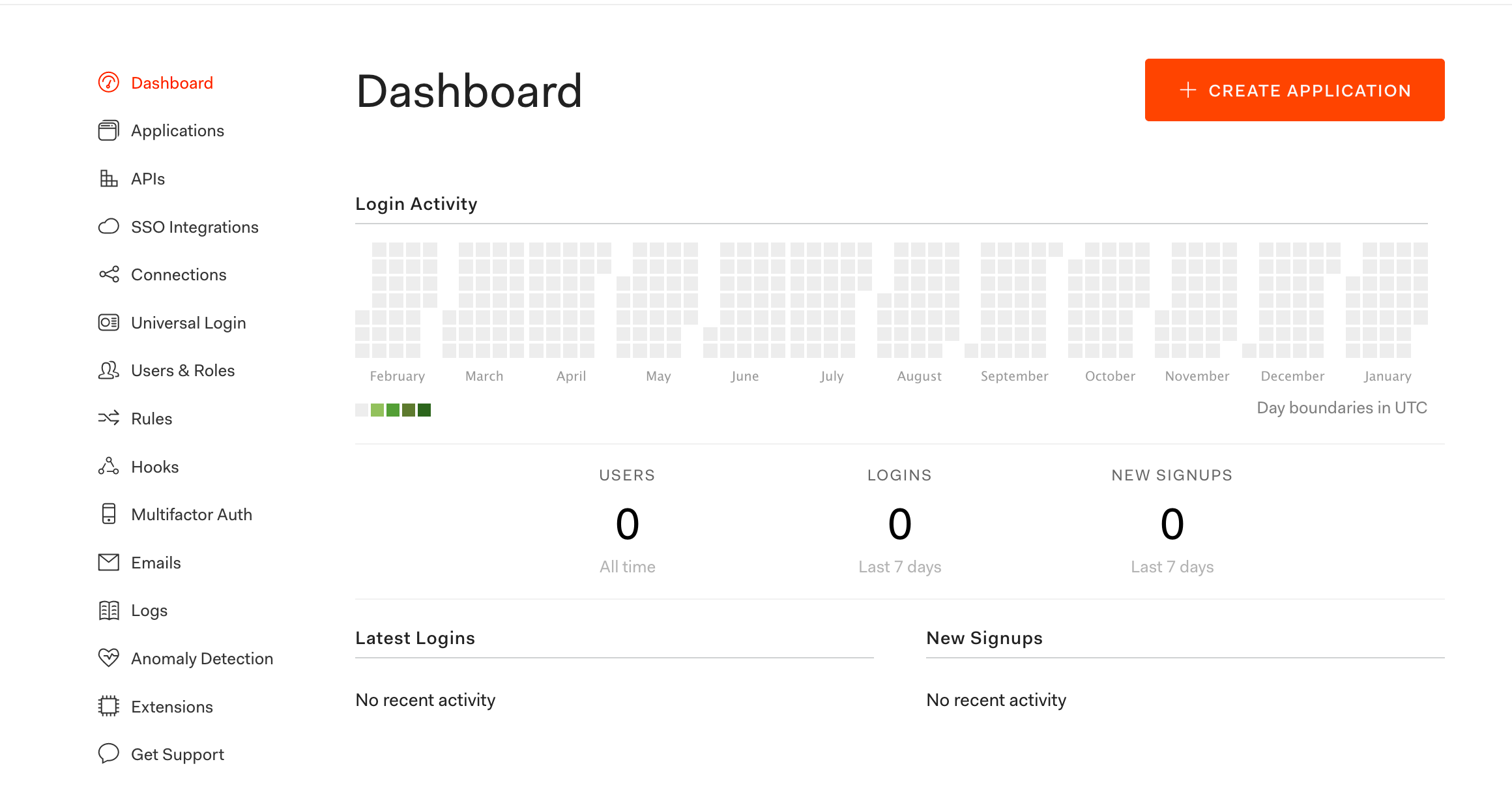
Now get the application keys and save them somewhere:
Go to the Applications > Defaut App > Settings.

Copy the Domain, Client ID, and Client Secret. They’ll be used in our Node app.
Step 2: Configure the Callback URL in Your Auth0 App
A callback URL is an URL in your application where Auth0 redirects the user after the authentication.
To add this, go to Applications > Defaut App > Settings, and
in the Allowed Callback URLs box, enter http://localhost:3000/callback
.
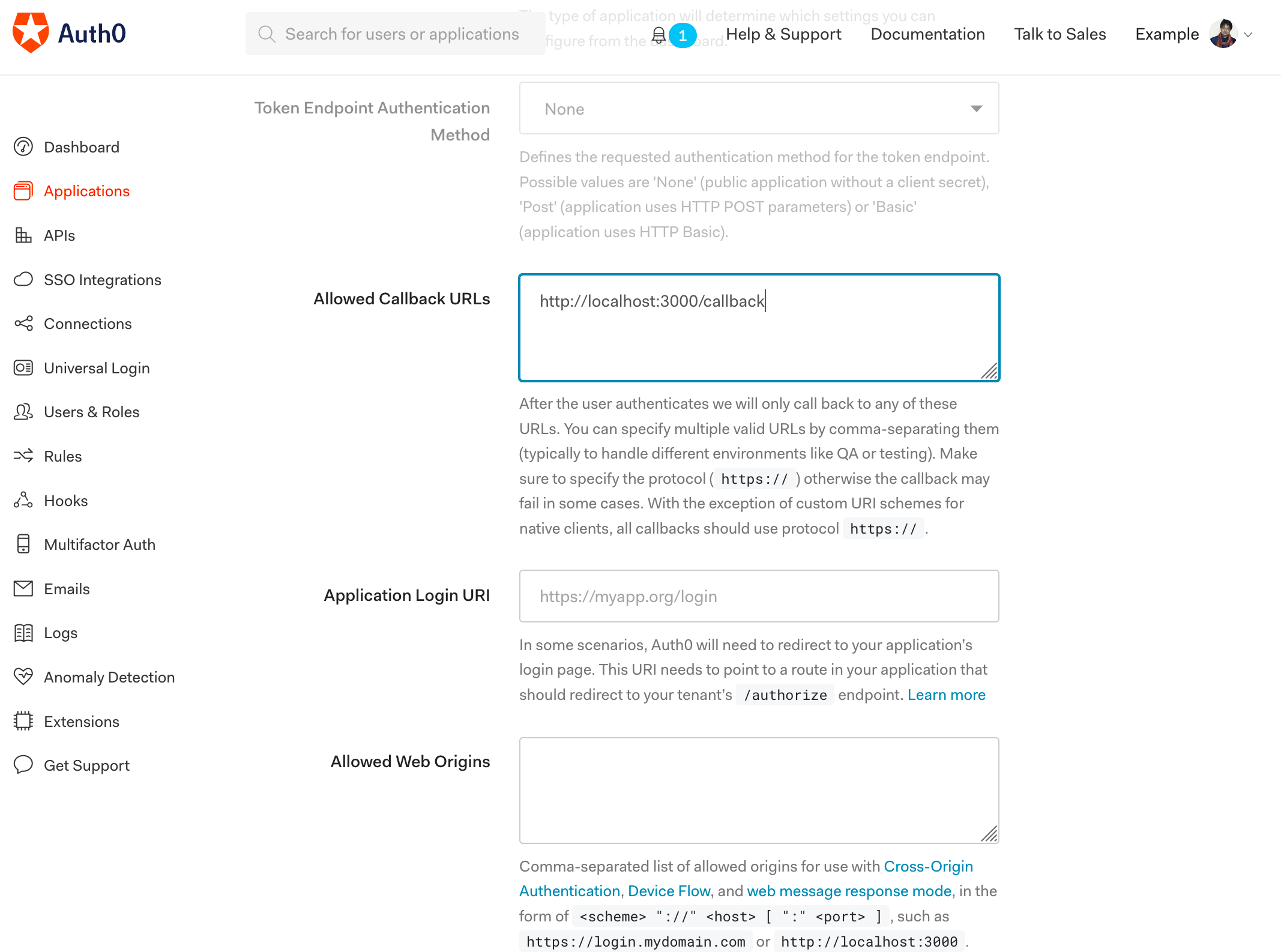
Step 3: Configure the Logout URL in Your Auth0 App
A logout URL is an URL in your application that Auth0 can return to after the user has been logged out of the authorization server. This is specified in the returnTo
query parameter.
To add this, go to Applications > Defaut App > Settings, and
in the Allowed Logout URLs box, enter http://localhost:3000
.
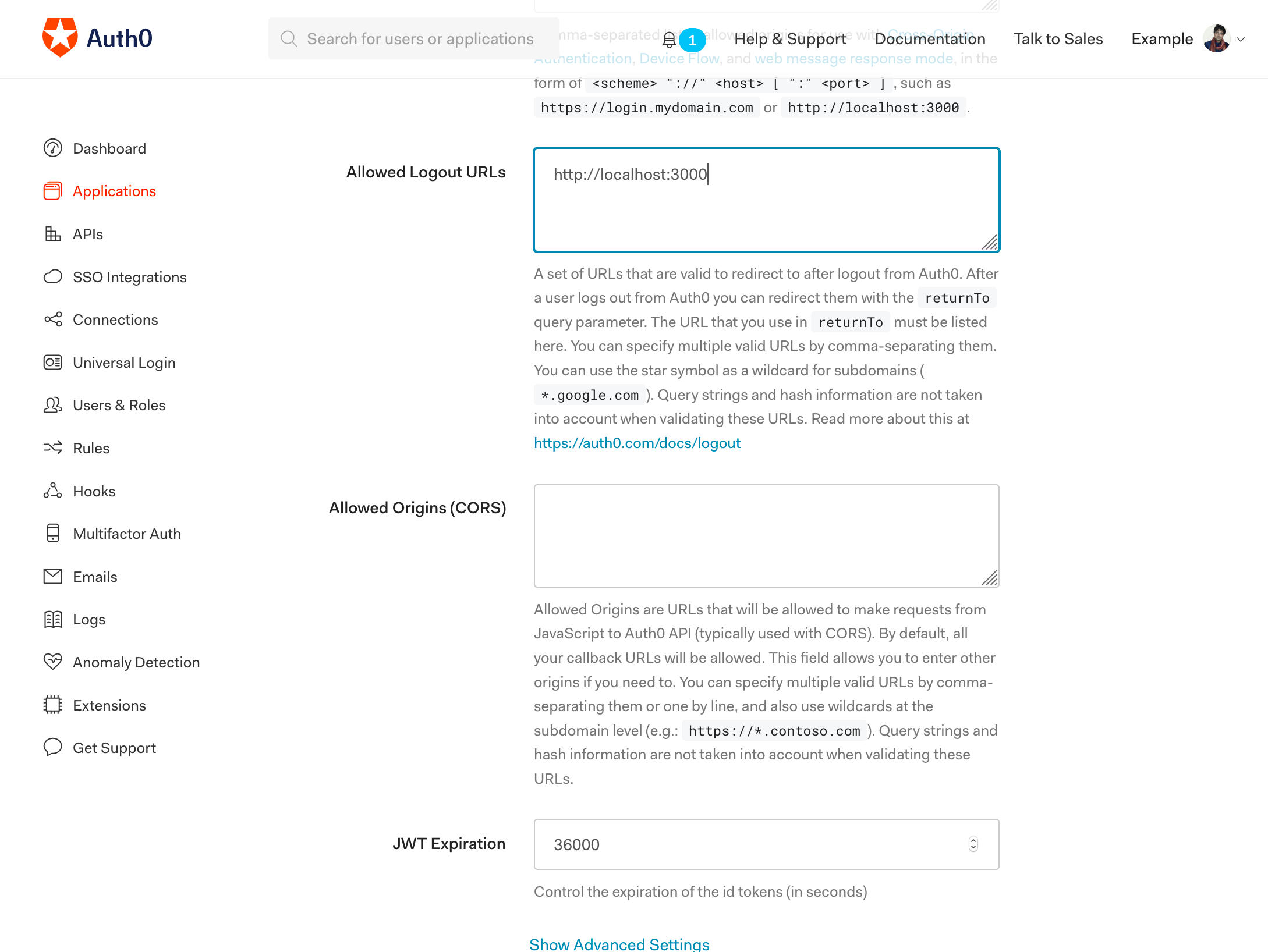
Step 4: Now Click on Save Changes
You need to save the changes you’ve done right now.

Step 5: Now Let’s Create a Node.js Server
To do this, create a folder called auth0
, and create a file named app.js
. Paste the following code inside app.js
:
var express = require('express');
var path = require('path');
var logger = require('morgan');
var cookieParser = require('cookie-parser');
var flash = require('connect-flash');
const app = express();app.get('/test',(req,res,next)=>{
res.send({message:"Server is on"});
})// View engine setup
app.set('views', path.join(__dirname, 'views'));
app.set('view engine', 'pug');app.use(logger('dev'));
app.use(cookieParser());
app.use(express.static(path.join(__dirname, 'public')));
app.use(flash());// Handle auth failure error messages
app.use(function (req, res, next) {
const err = new Error('Not Found');
err.status = 404;
next(err);
});app.use(function (err, req, res, next) {
res.status(err.status || 500);
res.send({message: err.message,error: {} });
});app.listen(8080,()=>{
console.log("Server is runnig on port 8080");
});module.exports = app;
Now open terminal, and go to the app.js
location. Run the command :
npm install express cookie-parser connect-flash morgan
After successfully running the above command, run the app.js
file using the below command:
node app.js
And you can see the output as:

Also, you can test your server by hitting the below API in your browser:

If you can see the above message, it means your server is running.
Now Let’s Configure Our Node.js App With Auth0
1: Create the .env file
Create the .env file in the root of your app, and paste the following code. Replace the actual values of YOUR_CLIENT_ID
, YOUR_DOMAIN
, and YOUR_CLIENT_SECRET
.
.env:
AUTH0_CLIENT_ID=YOUR_CLIENT_ID
AUTH0_DOMAIN=YOUR_DOMAIN
AUTH0_CLIENT_SECRET=YOUR_CLIENT_SECRET
2. Install the dependencies to support Auth0
We need to install the dependencies. To do so, run the following command:
npm install passport passport-auth0 express-session dotenv pug --save

npm install passport passport-auth0 express-session dotenv
pug --save
3. Add the following code into your app.js file
var session = require('express-session');
// config express-session
var sess = {
secret: 'CHANGE THIS TO A RANDOM SECRET',
cookie: {},
resave: false,
saveUninitialized: true
};
if (app.get('env') === 'production') {
// Use secure cookies in production (requires SSL/TLS)
sess.cookie.secure = true;
// Uncomment the line below if your application is behind a proxy (like on Heroku)
// or if you're encountering the error message:
// "Unable to verify authorization request state"
// app.set('trust proxy', 1);
}
app.use(session(sess));
4. Add the code for Passport and the application settings
// Load environment variables from .env
var dotenv = require('dotenv');
dotenv.config();
// Load Passport
var passport = require('passport');
var Auth0Strategy = require('passport-auth0');
// Configure Passport to use Auth0
var strategy = new Auth0Strategy(
{
domain: process.env.AUTH0_DOMAIN,
clientID: process.env.AUTH0_CLIENT_ID,
clientSecret: process.env.AUTH0_CLIENT_SECRET,
callbackURL:
process.env.AUTH0_CALLBACK_URL || 'http://localhost:3000/callback'
},
function (accessToken, refreshToken, extraParams, profile, done) {
// accessToken is the token to call Auth0 API (not needed in the most cases)
// extraParams.id_token has the JSON Web Token
// profile has all the information from the user
return done(null, profile);
}
);
passport.use(strategy);
app.use(passport.initialize());
app.use(passport.session());
Please make sure these last two commands are in your code after the application of the Express middleware (app.use(session(sess)
).
Now, your final app.js
file after the above code addition will look like this:
var express = require('express');
var path = require('path');
var logger = require('morgan');
var cookieParser = require('cookie-parser');
var session = require('express-session');
var dotenv = require('dotenv');
var passport = require('passport');
var Auth0Strategy = require('passport-auth0');
var flash = require('connect-flash');dotenv.config();// Configure Passport to use Auth0
var strategy = new Auth0Strategy({
domain: process.env.AUTH0_DOMAIN,
clientID: process.env.AUTH0_CLIENT_ID,
clientSecret: process.env.AUTH0_CLIENT_SECRET,
callbackURL:
process.env.AUTH0_CALLBACK_URL || 'http://localhost:3000/callback'
},function (accessToken, refreshToken, extraParams, profile, done) {
return done(null, profile);
});passport.use(strategy);// You can use this section to keep a smaller payload
passport.serializeUser(function (user, done) {
done(null, user);
});passport.deserializeUser(function (user, done) {
done(null, user);
});const app = express();
app.get('/test', (req, res, next) => {
res.send({ message: "Server is on" });
})// View engine setup
app.set('views', path.join(__dirname, 'views'));
app.set('view engine', 'pug');
app.use(logger('dev'));
app.use(cookieParser());// config express-session
var sess = {
secret: 'CHANGE THIS SECRET',
cookie: {},
resave: false,
saveUninitialized: true
};app.use(session(sess));
app.use(passport.initialize());
app.use(passport.session());
app.use(express.static(path.join(__dirname, 'public')));
app.use(flash());app.use(function (err, req, res, next) {
res.status(err.status || 500);|
res.send({message: err.message,error: err});
});app.listen(8080, () => {
console.log("Server is runnig on port 8080");
});
module.exports = app;
Step 6: Now We Need to Create the API Login
Now we need to create the API’s: /login
. Now we’ll create a folder called routes
at the root location with the following files:
routes/auth.js
to handle authenticationroutes/index.js
to serve the home page
Let's create a file called auth.js
inside the routes
folder and paste the following code:
auth.js:
// routes/auth.js
var express = require('express');
var router = express.Router();
var passport = require('passport');
var dotenv = require('dotenv');
dotenv.config();// Perform the login, after login Auth0 will redirect to callbackrouter.get('/login', passport.authenticate('auth0', {
scope: 'openid email profile'}), function (req, res) {
res.redirect('/');
});
module.exports = router;
Step 7: Create an Index Route to Serve the Homepage:
To do this, create a file called index.js
inside the routes
folder, and paste the following code:
// routes/index.js
var express = require('express');
var router = express.Router();/* GET home page. */router.get('/', function (req, res, next) {
res.render('layout', { title: 'Auth0 Webapp sample Nodejs' });|
});
module.exports = router;
Step 8: Add Routes Into Your app.js File
Paste the following code inside your app.js
file:
var authRouter = require('./routes/auth');
var indexRouter = require('./routes/index');
app.use('/', authRouter);
app.use('/', indexRouter);
Now your final app.js
file after the above code addition will look like this:
var express = require('express');
var path = require('path');
var logger = require('morgan');
var cookieParser = require('cookie-parser');
var session = require('express-session');
var dotenv = require('dotenv');
var passport = require('passport');
var Auth0Strategy = require('passport-auth0');
var flash = require('connect-flash');
var authRouter = require('./routes/auth');
var indexRouter = require('./routes/index');
dotenv.config();// Configure Passport to use Auth0
var strategy = new Auth0Strategy(
{
domain: process.env.AUTH0_DOMAIN,
clientID: process.env.AUTH0_CLIENT_ID,
clientSecret: process.env.AUTH0_CLIENT_SECRET,
callbackURL:process.env.AUTH0_CALLBACK_URL || 'http://localhost:3000/callback'
},
function (accessToken, refreshToken, extraParams, profile, done) {
return done(null, profile);
});passport.use(strategy);// You can use this section to keep a smaller payload
passport.serializeUser(function (user, done) {
done(null, user);
});passport.deserializeUser(function (user, done) {
done(null, user);
});const app = express();
app.get('/test', (req, res, next) => {
res.send({ message: "Server is on" });|
})// View engine setup
app.set('views', path.join(__dirname, 'views'));
app.set('view engine', 'pug');
app.use(logger('dev'));
app.use(cookieParser());// config express-session
var sess = {
secret: 'CHANGE THIS SECRET',
cookie: {},
resave: false,
saveUninitialized: true
};app.use(session(sess));
app.use(passport.initialize());
app.use(passport.session());
app.use(express.static(path.join(__dirname, 'public')));
app.use(flash());app.use('/', authRouter);
app.use('/', indexRouter);app.use(function (err, req, res, next) {
res.status(err.status || 500);
res.send({message: err.message,error: err});
});app.listen(8080, () => {
console.log("Server is runnig on port 8080");
});module.exports = app;
Step 9: Create Views for the Home Page:
To do this, create a folder called views
at the root location, and create a file named layout.pug
. Paste the following code:
doctype html
html
head
title= title
link(rel='stylesheet', href='/stylesheets/style.css')
link(rel='stylesheet', href='https://www.w3schools.com/w3css/4/w3.css')
link(rel='shortcut icon', href='//cdn2.auth0.com/styleguide/latest/lib/logos/img/favicon.png')
body
nav.w3-bar.w3-border.w3-light-grey( role="navigation" )
.w3-bar-item.w3-text-grey Auth0 - NodeJS
a(href="/").w3-bar-item.w3-button Home
a(id="qsLoginBtn" href="/login").w3-bar-item.w3-button Log In
block content
h4 Welcome in Auth0 Implementation: Shubham Verma blogs
Let’s see the snapshot:

layout.pug
Step 10: Run the App, and See the Result:
Now that you’ve done your coding, it’s time to run the app.js
file using the following command:
node app.js
You can see the output is:

Now open the browser, and enter the URL localhost:8080
. And see the page:
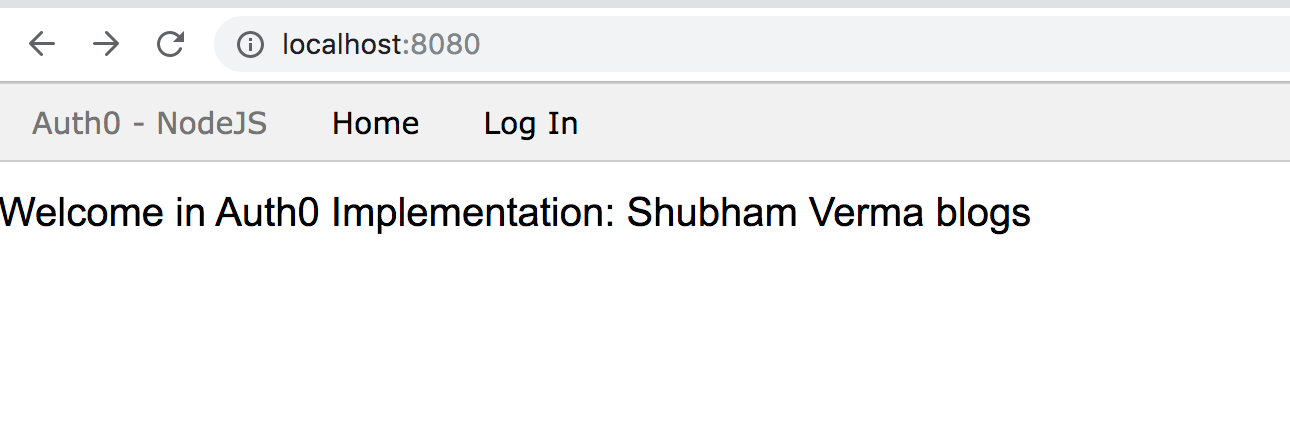
Now click Log In, and see the magic. You can see the Auth0 login page displayed.

Let’s see the combined result in the following GIF:
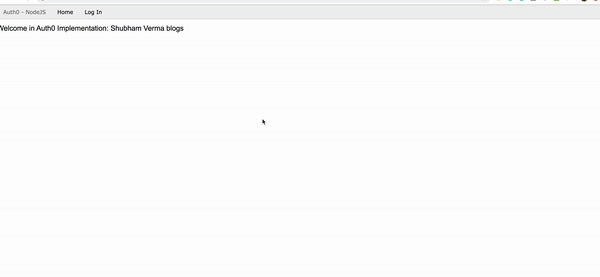
Congratulations. Now we’ve implemented Auth0 with Node.js.
To understand more about Node.js, read this
Comments
Post a Comment